How to setup caching?
Click your Service Bus, Access Control & Caching tab on the left side of the Windows Azure portal. Click New Namespace from the toolbar and create the one you like and choose the appropriate size. See below.
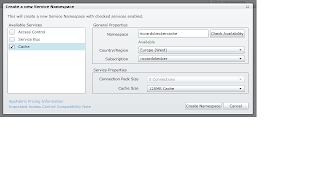
After creation everything is arranged by the platform. Security, scalability, availability, access tokens etc.
After you created the Caching Namespace you are able to start using it. First of all add the correct references to the assemblies involved in Caching. You can find them in the Program Files\Windows Azure AppFabric SDK\V1.0\Assemblies\NET4.0\Cache folder. Select the Caching.Client and Caching.Core assemblies and voila. ASP.NET project also need the Microsoft.Web.DistributedCache assembly.
The easiest way to create access to your cache is to copy the configuration to you app.config (or web.config). Click the namespace of the cache in Azure Portal. Then click in the toolbar on View Client Configuration on copy the settings and paste them in your config file in visual studio. The settings look like this.
Create a consoleapp and copy code as below and voila, your scalable, highly available, cloudy cache is actually up and running!
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using Microsoft.ApplicationServer.Caching;
using Microsoft.ApplicationServer;
namespace ConsoleApplication1
{
class Program
{
static void Main(string[] args)
{
// Cache client configured by settings in application configuration file.
DataCacheFactory cacheFactory = new DataCacheFactory();
DataCache defaultCache = cacheFactory.GetDefaultCache();
// Add and retrieve a test object from the default cache.
defaultCache.Add("myuniquekey", "testobject");
string strObject = (string)defaultCache.Get("testkey");
}
}
}
What can you store in Cache? Actually everything as long as it's serializable.
Have fun with it and use the features of the Windows Azure platform!
Only downside to Azure AppFabric Caching is that you don't have access to L2 and L1 level CPU cache. On a dedicated server in Windows 2008 R2 you do.
ReplyDeletePlus in my opinion Azure AppFabric caching is expensive and costs need to drop 50%.
Have you done any measurements on how much this would speed up Azure SQL usage?
ReplyDeleteAntti Makkonen
@anttimakkonen
Antti,
ReplyDeletedidn't do any actual benchmarking but it will speed your application up because you can reduce the roundtrips to your SQL (Azure) database. So fixed data like lookuptables are good candidates to cache.
Hope this helps!